How can we help?
Оригинал статьи здесь. Содержимое файла sample.csv:
id,name,description,createdAt 1,Node.js,JavaScript runtime environment,2019-09-03 2,Vue.js,JavaScript Framework for building UI,2019-09-06 3,Angular.js,Platform for building mobile & desktop web app,2019-09-09
Создаем таблицу, для чего подключаемся к базе:
PS C:\node.js\csvToPostgres2> psql.exe -d postgres -U postgres Пароль пользователя postgres: psql (14.2) ПРЕДУПРЕЖДЕНИЕ: Кодовая страница консоли (866) отличается от основной страницы Windows (1251). 8-битовые (русские) символы могут отображаться некорректно. Подробнее об этом смотрите документацию psql, раздел "Notes for Windows users". Введите "help", чтобы получить справку. postgres=# CREATE TABLE category postgres(# id integer NOT NULL, postgres(# name VARCHAR(255) NOT NULL, postgres(# description VARCHAR(255), postgres(# created_at DATE); CREATE TABLE
Смотрим содержимое таблицы — \d category:

Импортируем модули fast-csv и pg:
npm install fast-csv pg
Ну и собственно сам код:
const fs = require("fs"); const fastcsv = require("fast-csv"); let stream = fs.createReadStream("sample.csv"); let csvData = []; let csvStream = fastcsv .parse() .on("data", function(data) { csvData.push(data); }) .on("end", function() { // remove the first line: header csvData.shift(); // connect to the PostgreSQL database // save csvData }); stream.pipe(csvStream); const Pool = require("pg").Pool; // remove the first line: header csvData.shift(); // create a new connection pool to the database const pool = new Pool({ host: "localhost", user: "postgres", database: "postgres", password: "postgres", port: 5432 }); const query = "INSERT INTO category (id, name, description, created_at) VALUES ($1, $2, $3, $4)"; pool.connect((err, client, done) => { if (err) throw err; try { csvData.forEach(row => { client.query(query, row, (err, res) => { if (err) { console.log(err.stack); } else { console.log("inserted " + res.rowCount + " row:", row); } }); }); } finally { done(); } });
Запускаем код видим как наполняется таблица:
PS C:\node.js\csvToPostgres2> node .\index.js inserted 1 row: [ '1', 'Node', "'JavaScript runtime environment'", '2019-09-03' ] inserted 1 row: [ '2', 'Vue', "'JavaScript Framework for building UI'", '2019-09-06' ] inserted 1 row: [ '3', 'Angular', "'Platform for building mobile & desktop web app'", '2019-09-09' ]
Смотрим содержимое таблицы SELECT * FROM category:
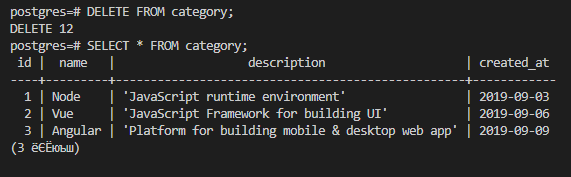
Очистить содержимое таблицы: DELETE FROM category;